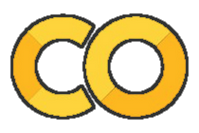
Memory, base class¶
This implements the Cassandra-specific I/O facilities, and is then used by LangChain to power every other, more sophisticated, abstraction around keeping chat memory.
In [1]:
Copied!
from langchain.memory import CassandraChatMessageHistory
from langchain.memory import CassandraChatMessageHistory
In [2]:
Copied!
from cqlsession import getCQLSession, getCQLKeyspace
cqlMode = 'astra_db' # 'astra_db'/'local'
session = getCQLSession(mode=cqlMode)
keyspace = getCQLKeyspace(mode=cqlMode)
from cqlsession import getCQLSession, getCQLKeyspace
cqlMode = 'astra_db' # 'astra_db'/'local'
session = getCQLSession(mode=cqlMode)
keyspace = getCQLKeyspace(mode=cqlMode)
Create the ChatMessageHistory¶
In [3]:
Copied!
message_history = CassandraChatMessageHistory(
session_id='test-session',
session=session,
keyspace=keyspace,
ttl_seconds = 3600,
)
message_history.clear()
message_history = CassandraChatMessageHistory(
session_id='test-session',
session=session,
keyspace=keyspace,
ttl_seconds = 3600,
)
message_history.clear()
The memory starts empty:
In [4]:
Copied!
message_history.messages
message_history.messages
Out[4]:
[]
Insert and retrieve chat messages¶
In [5]:
Copied!
message_history.add_user_message('Hello, I am human.')
message_history.add_user_message('Hello, I am human.')
In [6]:
Copied!
message_history.add_ai_message('Hello, I am the bot.')
message_history.add_ai_message('Hello, I am the bot.')
In [7]:
Copied!
message_history.messages
message_history.messages
Out[7]:
[HumanMessage(content='Hello, I am human.', additional_kwargs={}, example=False), AIMessage(content='Hello, I am the bot.', additional_kwargs={}, example=False)]
In [8]:
Copied!
message_history.clear()
message_history.clear()
In [9]:
Copied!
message_history.messages
message_history.messages
Out[9]:
[]